서문
Brightcove의 Pulse 플러그인을 사용하면 Invidi의 Pulse SDK를 Android 용 Brightcove Native SDK와 통합 할 수 있습니다. Pulse는 동영상 광고 플랫폼입니다. 캠페인 및 구성에 대한 자세한 내용은사용자 설명서 .
단계
Pulse 플랫폼에서 캠페인이 생성되면 Android 용 Brightcove 네이티브 SDK 용 Pulse 플러그인 사용을 시작할 수 있습니다. Pulse 플러그인을 프로젝트와 통합하려면 다음 단계를 따르십시오.
-
모듈에서 build.gradle파일에 Pulse 플러그인 종속성을 추가합니다.
dependencies { implementation 'com.brightcove.player:android-pulse-plugin:6.12.0' }
-
다운로드 Pulse SDK .aar 파일 .
-
당신의앱 / libs폴더를 열고 build.gradle모듈의 파일. 다음을 수정하십시오.
dependencies { implementation fileTree(dir: 'libs', include: ['*.jar', '*.aar']) }
-
당신의 MainActivity.java파일을 인스턴스화하여 Pulse 플러그인을 초기화합니다.
PulseComponent
캠페인 용으로 생성 된 Pulse 호스트 URL로@Override protected void onCreate(Bundle savedInstanceState) { // ... // Creating pulse component PulseComponent pulseComponent = new PulseComponent( "your pulse host url", brightcoveVideoView.getEventEmitter(), brightcoveVideoView); // ... }
-
설정
PulseComponent
경청자.pulseComponent.setListener(new PulseComponent.Listener() { @NonNull @Override public PulseSession onCreatePulseSession( @NonNull String hostUrl, @NonNull Video video, @NonNull ContentMetadata contentMetadata, @NonNull RequestSettings requestSettings) { // See step 3a return Pulse.createSession(contentMetadata, requestSettings); } @Override public void onOpenClickthrough(@NonNull PulseVideoAd pulseVideoAd) { } });
-
구현
onCreatePulseSession
생성하는 방법,PulseSession
그리고 그것을PulseComponent
. 세 가지 매개 변수가 있습니다.- 펄스 호스트
- 콘텐츠 메타 데이터 설정
- 요청 설정
@NonNull @Override public PulseSession onCreatePulseSession( @NonNull String hostUrl, @NonNull Video video, @NonNull ContentMetadata contentMetadata, @NonNull RequestSettings requestSettings) { // Set the pulse Host: Pulse.setPulseHost(pulseHostUrl, null, null); // Content metadata settings contentMetadata.setCategory("skip-always"); contentMetadata.setTags(Collections.singletonList("standard-linears")); contentMetadata.setIdentifier("demo"); // Request Settings: // Adding mid-rolls List<Float> midrollCuePoints = new ArrayList<>(); midrollCuePoints.add(60f); requestSettings.setLinearPlaybackPositions(midrollCuePoints); // Create and return the PulseSession return Pulse.createSession(contentMetadata, requestSettings); }
-
구현
onOpenClickthrough
메소드, 더 알아보기선형 광고에서 버튼을 클릭합니다. 이 콜백의 일반적인 작업은 예상 URL로 브라우저를 여는 것입니다.@Override public void onOpenClickthrough(@NonNull PulseVideoAd pulseVideoAd) { Intent intent = new Intent(Intent.ACTION_VIEW) .setData(Uri.parse(pulseVideoAd.getClickthroughURL().toString())); brightcoveVideoView.getContext().startActivity(intent); pulseVideoAd.adClickThroughTriggered(); }
-
콘텐츠 재생
Catalog catalog = new Catalog.Builder( eventEmitter, getString(R.string.account)) .setPolicy(getString(R.string.policy)) .build(); catalog.findVideoByID(getString(R.string.videoId), new VideoListener() { // Add the video found to the queue with add(). // Start playback of the video with start(). @Override public void onVideo(Video video) { brightcoveVideoView.add(video); brightcoveVideoView.start(); } });
펄스 일시 중지 광고
Pulse 캠페인에 '광고 일시 중지'가 구성되어 있으면 콘텐츠가 일시 중지 될 때 Pulse 플러그인이 사용자에게 표시됩니다.
오류 처리
모든 오류는 아래와 같이 EventType.AD_ERROR 이벤트를 사용하여 개발자에게 표시됩니다.
eventEmitter.on(EventType.AD_ERROR, event -> {
Throwable error = event.getProperty(Event.ERROR, Throwable.class);
Log.e(TAG, "AD_ERROR: ", error);
});
UI 사용자 지정
내부적으로 Pulse 플러그인은PulseAdView
사용하여R.layout.pulse_ad_view
레이아웃 ID. 다른 레이아웃의 경우 동일한 이름의 레이아웃 파일을 생성하여해상도 / 레이아웃예배 규칙서. 이것은 기본 레이아웃을 재정의합니다.
기본값을 바꾸려면 다음 ID를 사용하십시오.
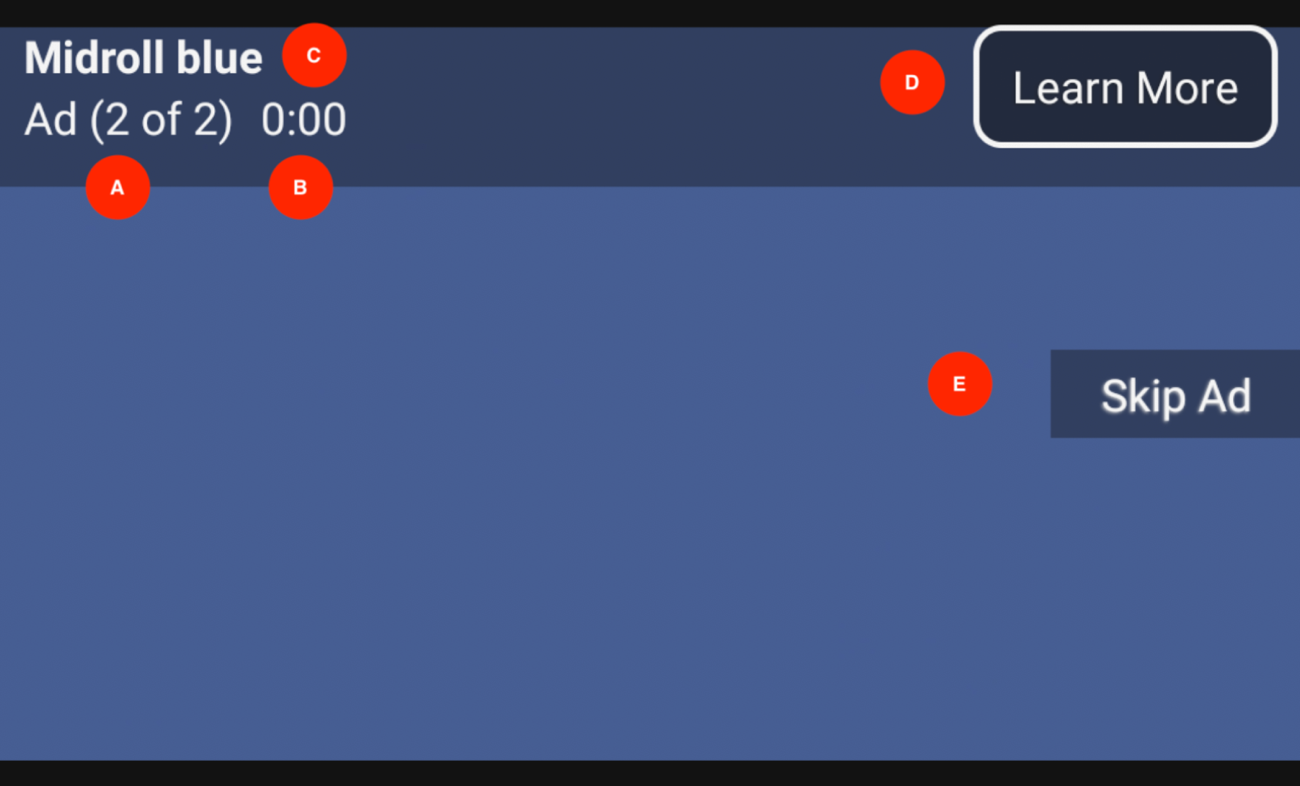
인덱스 | 보기 유형 | ID보기 |
---|---|---|
A | 텍스트 뷰 | pulse_ad_number_view |
B | 텍스트 뷰 | pulse_ad_countdown_view |
C | 텍스트 뷰 | pulse_ad_name_view |
D | 텍스트 뷰 | pulse_ad_learn_more_view |
E | 텍스트 뷰 | pulse_skip_ad_view |
완전한 코드 샘플
다음은 Android 용 Native SDK와 함께 Pulse 플러그인을 사용하기위한 전체 코드 샘플입니다.
활동
다음은 전체 활동 코드의 예입니다.
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
setContentView(R.layout.activity_main);
final BrightcoveVideoView videoView = findViewById(R.id.video_view);
super.onCreate(savedInstanceState);
EventEmitter eventEmitter = videoView.getEventEmitter();
// Pulse setup
PulseComponent pulseComponent = new PulseComponent(
"https://pulse-demo.videoplaza.tv",
eventEmitter,
videoView);
pulseComponent.setListener(new PulseComponent.Listener() {
@NonNull
@Override
public PulseSession onCreatePulseSession(
@NonNull String pulseHostUrl,
@NonNull Video video,
@NonNull ContentMetadata contentMetadata,
@NonNull RequestSettings requestSettings) {
Pulse.setPulseHost(pulseHostUrl, null, null);
contentMetadata.setCategory("skip-always");
contentMetadata.setTags(Collections.singletonList("standard-linears"));
contentMetadata.setIdentifier("demo");
// Adding mid-rolls
List<Float> midrollCuePoints = new ArrayList<>();
midrollCuePoints.add(60f);
requestSettings.setLinearPlaybackPositions(midrollCuePoints);
return Pulse.createSession(
contentMetadata,
requestSettings);
}
@Override
public void onOpenClickthrough(@NonNull PulseVideoAd ad) {
Intent intent = new Intent(Intent.ACTION_VIEW)
.setData(Uri.parse(ad.getClickthroughURL().toString()));
videoView.getContext().startActivity(intent);
ad.adClickThroughTriggered();
}
});
Catalog catalog = new Catalog.Builder(eventEmitter, "YourAccountId")
.setPolicy("YourPolicyKey")
.build();
catalog.findVideoByID("YourVideoId", new VideoListener() {
// Add the video found to the queue with add().
// Start playback of the video with start().
@Override
public void onVideo(Video video) {
videoView.add(video);
videoView.start();
}
});
}
}
레이아웃
다음은 레이아웃 코드의 예입니다. R.layout.pulse_ad_view
.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<RelativeLayout
android:id="@+id/view_ad_details"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@drawable/pulse_skip_button_background_selector">
<TextView
android:id="@+id/pulse_ad_name_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_marginTop="4dp"
android:paddingTop="4dp"
android:paddingStart="8dp"
android:paddingEnd="8dp"
android:textColor="@color/white"
android:background="@color/bmc_live"
android:textStyle="bold"
tools:text="Preroll blue"/>
<TextView
android:id="@+id/pulse_ad_number_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingStart="8dp"
android:paddingEnd="4dp"
android:paddingBottom="4dp"
android:layout_marginBottom="8dp"
android:layout_below="@id/pulse_ad_name_view"
android:textColor="@color/white"
android:background="@color/white_semi_trans"
tools:text="Ad (1 of 2)"/>
<TextView
android:id="@+id/pulse_ad_countdown_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingStart="4dp"
android:paddingEnd="4dp"
android:paddingBottom="4dp"
android:layout_marginBottom="4dp"
android:layout_below="@id/pulse_ad_name_view"
android:layout_toEndOf="@+id/pulse_ad_number_view"
android:textColor="@color/green_almost_opaque"
android:text=""
tools:text="00:06"/>
<TextView
android:id="@+id/pulse_ad_learn_more_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="@dimen/pulse_ad_learn_more_margin_left"
android:layout_marginTop="@dimen/pulse_ad_learn_more_margin_top"
android:layout_marginEnd="@dimen/pulse_ad_learn_more_margin_right"
android:layout_marginBottom="@dimen/pulse_ad_learn_more_margin_bottom"
android:layout_alignTop="@id/pulse_ad_name_view"
android:layout_alignBottom="@id/pulse_ad_countdown_view"
android:layout_alignParentEnd="true"
android:background="@drawable/pulse_learn_more_button_background"
android:paddingStart="12dp"
android:paddingEnd="12dp"
android:padding="@dimen/pulse_ad_learn_more_padding_default"
android:gravity="center"
android:shadowColor="@color/brightcove_semitransparent"
android:shadowDx="-1"
android:shadowDy="1"
android:shadowRadius="1.5"
android:text="@string/pulse_message_learn_more"
android:textColor="@color/pulse_button_text_color"
android:nextFocusUp="@id/pulse_skip_ad_view"
android:textSize="@dimen/pulse_message_text_size"
android:visibility="gone"
tools:visibility="visible" />
</RelativeLayout>
<TextView
android:id="@+id/pulse_skip_ad_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:maxWidth="164dp"
android:layout_alignParentEnd="true"
android:layout_centerVertical="true"
android:layout_marginBottom="@dimen/pulse_skip_ad_margin_bottom"
android:background="@drawable/pulse_skip_button_background_selector"
android:ellipsize="none"
android:gravity="center"
android:maxLines="2"
android:paddingStart="@dimen/pulse_skip_ad_padding_left"
android:paddingEnd="@dimen/pulse_skip_ad_padding_right"
android:paddingTop="@dimen/pulse_skip_ad_padding"
android:paddingBottom="@dimen/pulse_skip_ad_padding"
android:scrollHorizontally="false"
android:shadowColor="@color/brightcove_shadow"
android:shadowDx="-1"
android:shadowDy="1"
android:shadowRadius="1.5"
android:text="@string/pulse_message_skip_ad"
android:textColor="@color/pulse_button_text_color"
android:textSize="@dimen/pulse_message_text_size"
android:visibility="gone"
android:nextFocusUp="@id/pulse_ad_learn_more_view"
android:focusable="true"
tools:visibility="visible"/>
</RelativeLayout>